How to Integrate OpenAI API Across Diverse Applications
by Andrej Avbelj, Software Engineer
1. Leveraging OpenAI's API Across Diverse Applications
The integration of advanced artificial intelligence capabilities into applications is no longer a futuristic idea but a present-day reality. OpenAI's powerful APIs, including models like GPT-4, offer vast possibilities for enhancing application features across various industries. This blog post will explore how different sectors can leverage OpenAI's API to improve user experiences, streamline operations, and drive innovation.
2. Overview of OpenAI's API
OpenAI's API provides access to advanced language models that can understand and generate human-like text based on the input they receive. These models are trained on a diverse range of internet text, but unlike most AI models, they can perform a wide variety of tasks without task-specific training. This versatility makes OpenAI's API incredibly powerful for numerous applications, from simple chatbots to complex data analysis systems.
3. Integration across Different Sectors
3.1. E-Commerce
Enhanced Customer Service
E-commerce platforms can use the API to power chatbots that handle customer inquiries, track orders, and provide product recommendations. By understanding natural language, these bots can deliver a more human-like interaction, improving customer satisfaction.
Personalized Shopping Experience
AI can analyze browsing patterns and purchase history to offer personalized shopping experiences. OpenAI's API can generate product descriptions, recommend products, and even help in curating personalized marketing messages.
3.2. Healthcare
Symptom Assessment
Integrating the API into telehealth platforms allows for preliminary symptom assessment before a patient consults with a doctor. By providing a description of their symptoms, patients can receive immediate feedback on possible conditions and advice on the next steps.
Medical Research
Researchers can use the API to digest large volumes of medical texts, research papers, and clinical trials, summarizing information and even suggesting areas for further study.
3.3. Education
Personalized Learning
Educational apps can harness the API to create adaptive learning systems that respond to the needs of individual students, offer explanations, solve problems, and provide practice exercises in a conversational manner.
Automated Content Creation
Teachers and content creators can generate educational content, including customized reading materials, quizzes, and summaries to aid learning.
3.4. Finance
Automated Financial Advice
Financial institutions can use the API to provide personalized financial advice, investment recommendations, and budgeting tips based on individual financial goals and risk tolerance.
Fraud Detection
By analyzing transaction data and customer behavior, the API can help detect fraudulent activities and prevent unauthorized access to accounts.
3.5 Media & Entertainment
Personalized Content Recommendations
Media platforms can leverage the API to analyze user preferences, viewing habits, and social media interactions to recommend personalized content, such as movies, TV shows, music, and articles.
Content Creation
Journalists, bloggers, and content creators can use the API to generate engaging headlines, articles, and social media posts, saving time and enhancing creativity.
Enough of the theory! Let's dive into a practical example of integrating OpenAI's API into a healthcare application to enhance patient communication.
4. Example Use Case: Integrating OpenAI's API into a Healthcare Application for Enhanced Patient Communication
The healthcare industry continually seeks innovative solutions to improve patient care and streamline provider workflows. One such innovation is the integration of AI-powered tools like OpenAI's API into healthcare applications. This blog post explores a detailed example of how OpenAI's API can be used to enhance doctor-patient communications by automating the initial assessment and response process regarding patient symptoms.
4.1. Overview
The integration of OpenAI's API into a healthcare application can revolutionize how patients interact with their healthcare providers. By automating the initial analysis of symptoms and generating preliminary medical advice, the system can save time for doctors and provide immediate feedback to patients. This process not only speeds up the healthcare delivery but also allows doctors to focus more on critical cases and personalized patient care.
Let's say we have already implemented web application functionality that allows patients to input their symptoms and receive response from the Doctor that needs to manually analyze the symptoms and provide feedback. We can enhance this system by integrating OpenAI's API to automate the symptom analysis and generate preliminary advice for the patient.
4.2. Integration Steps
4.2.1. OpenAI API Setup
First, you need to sign up for OpenAI's API and obtain an API key. This key will be used to authenticate your requests to the API.
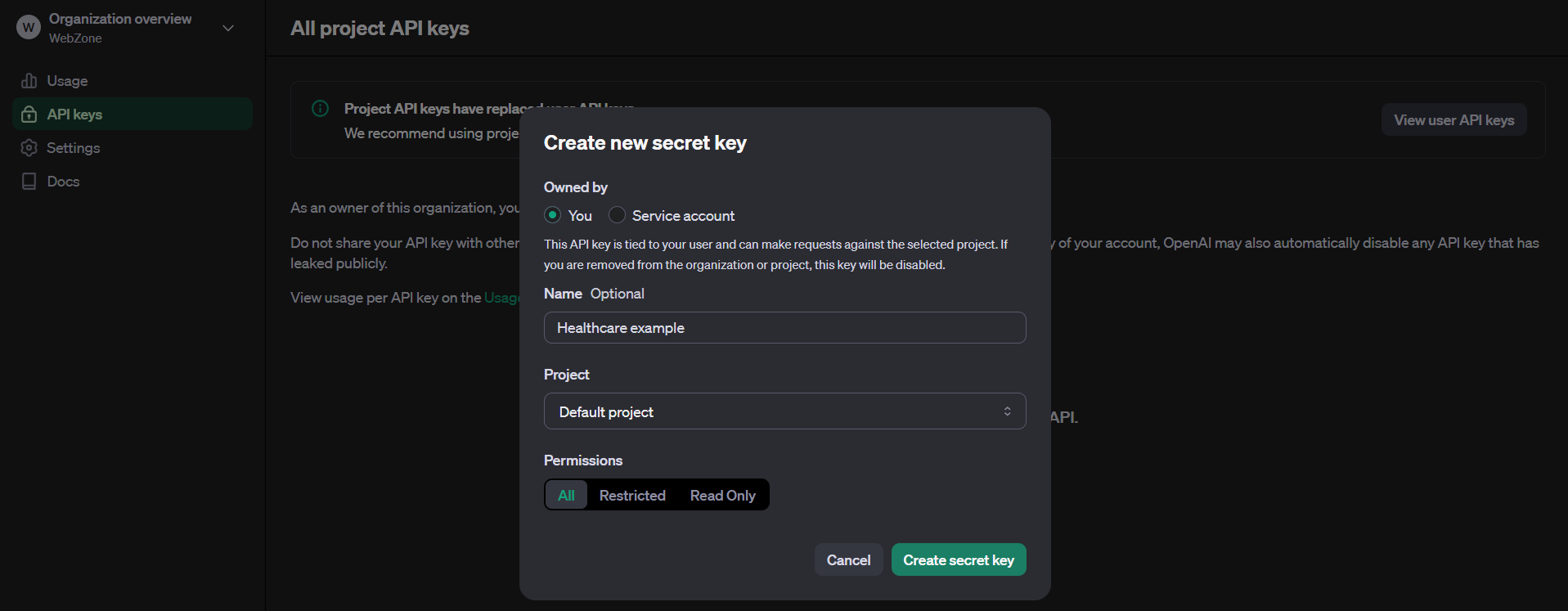
Copy the API key and securely store it for future use.
4.2.2. Implementing the Integration
Let's say you have backend developed in Node.js. You can use the axios
library to make HTTP requests to OpenAI's API.
Firstly store the OpenAI API key in a secure environment variable (.env file).
OPENAI_API_KEY=your-api-key-here
For the sake of the example, we will include everything in a single file, but in a real-world scenario, you would separate the API call into a separate module.
const express = require('express');
const bodyParser = require('body-parser');
const axios = require('axios');
const baseOpenAIUrl = 'https://api.openai.com/v1/chat/completions';
const modelOpenAIUrl = 'gpt-3.5-turbo-0125'; // Define the model to use
const diagnosisSchema = ''; // Define the schema for the diagnosis response, e.g., JSON schema
const openAIOptions = {
headers: {
'Authorization': `Bearer ${process.env.OPENAI_API_KEY}`,
'Content-Type': 'application/json'
}
};
const app = express();
app.use(bodyParser.json());
app.post('/analyze-symptoms', async (req, res) => {
try {
const patientSymptoms = req.body.symptoms;
const medicalAdvice = await getMedicalAdvice(patientSymptoms);
res.json(medicalAdvice);
} catch (error) {
console.error('Error processing the patient symptoms:', error);
res.status(500).send('An error occurred while processing your request.');
}
});
const getMedicalAdvice = async (symptoms) => {
const prompt = `Generate medical advice in JSON schema based on the following symptoms: ${symptoms}`;
const payload = {
model: modelOpenAIUrl,
messages: [
{ role: "system", content: "You are a helpful AI doctor assistant and you return reponses in JSON format." },
{ role: "user", content: prompt }],
response_format: { type: "json_object" }, // Tell the API to return JSON
functions: [{ name: "set_diagnosis", body: diagnosisSchema }], // Define the function to set the diagnosis
function_call: {name: "set_diagnosis"}, // Call the function to set the diagnosis
temperature: 0.7, // Define the temperature for sampling
max_tokens: 2000, // Define the maximum number of tokens for the response
frequency_penalty: 0.0, // Define the frequency penalty
presence_penalty: 0.0 // Define the presence penalty
};
return callOpenAI(payload);
};
const callOpenAI = async (payload) => {
try {
const response = await axios.post(baseOpenAIUrl, payload, openAIOptions);
const data = JSON.parse(response.data.choices[0].message.content);
// Implement parsing logic here based on expected response format
if (data.choices && data.choices.length > 0 && data.choices[0].message.content && data.choices[0].message.content.function_call) {
return JSON.parse(data.choices[0].message.content.function_call.arguments);
} else {
throw new Error('Invalid response from OpenAI API.');
}
} catch (error) {
console.error('Error calling OpenAI API:', error);
throw new Error('An error occurred while calling OpenAI API.');
}
};
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
In this example, we define an endpoint /analyze-symptoms
that receives the patient's symptoms, generates a prompt for the OpenAI API, and processes the response to return the medical advice in JSON format. The getMedicalAdvice
function constructs the payload for the API request, while the callOpenAI
function makes the HTTP request to the API and processes the response.
We need to define JSON schema for the diagnosis response otherwise we will hardly get any useful information from the API. The schema should describe each parameters by the type and the description.
Into the diagnosisSchema
variable we should put the following JSON schema:
{
"type": "object",
"description": "This schema defines the structure for a detailed medical response, including diagnostic insights, treatment suggestions, and educational resources based on patient symptoms.",
"properties": {
"introduction": {
"type": "string",
"description": "A brief introduction to the response provided to the patient, setting the context for the information that follows."
},
"possible_conditions": {
"type": "array",
"description": "A list of medical conditions that could match the patient's symptoms, along with confidence levels and descriptions for each.",
"items": {
"type": "object",
"properties": {
"condition": {
"type": "string",
"description": "The name of the possible medical condition."
},
"confidence": {
"type": "number",
"description": "The confidence level (from 0 to 1) representing the likelihood of this condition being a match for the symptoms described."
},
"description": {
"type": "string",
"description": "A detailed description of the medical condition."
}
},
"required": ["condition", "confidence", "description"]
}
},
"recommended_treatments": {
"type": "array",
"description": "Recommended treatments and medications for the diagnosed condition, including detailed instructions and precautions.",
"items": {
"type": "object",
"properties": {
"treatment": {
"type": "string",
"description": "A general description of the treatment or care recommendation."
},
"medications": {
"type": "array",
"description": "A list of medications recommended, including dosages and precautions.",
"items": {
"type": "object",
"properties": {
"name": {
"type": "string",
"description": "The name of the medication."
},
"dosage": {
"type": "string",
"description": "Recommended dosage instructions for the medication."
},
"precautions": {
"type": "string",
"description": "Specific precautions or warnings related to the use of the medication."
}
},
"required": ["name", "dosage", "precautions"]
}
},
"instructions": {
"type": "string",
"description": "Additional instructions or recommendations for self-care or treatment application."
}
},
"required": ["treatment"]
}
},
"advice": {
"type": "string",
"description": "General advice or next steps for the patient, including when to seek further medical attention."
},
"follow_up": {
"type": "object",
"description": "Suggestions for follow-up actions, including questions for the patient to answer that could help further refine the diagnosis or treatment.",
"properties": {
"questions": {
"type": "array",
"description": "A list of follow-up questions to be answered by the patient.",
"items": {
"type": "string"
}
},
"instructions": {
"type": "string",
"description": "Instructions to the patient on how to proceed with the follow-up."
}
},
"required": ["questions", "instructions"]
},
"additional_resources": {
"type": "array",
"description": "Links to additional resources that provide further information about the medical conditions mentioned.",
"items": {
"type": "object",
"properties": {
"condition": {
"type": "string",
"description": "The medical condition related to the resource."
},
"url": {
"type": "string",
"description": "URL to the resource providing more detailed information about the condition."
}
},
"required": ["condition", "url"]
}
},
"closing": {
"type": "string",
"description": "A closing statement to end the response, reinforcing the commitment to the patient's health and encouraging them to stay informed."
}
},
"required": ["introduction", "possible_conditions", "recommended_treatments", "advice", "follow_up", "additional_resources", "closing"]
}
This schema defines the structure for a detailed medical response, including diagnostic insights, treatment suggestions, and educational resources based on patient symptoms.
We could parse the response and return the structured medical advice to the patient, including possible conditions, recommended treatments, advice, follow-up instructions, and additional resources.
4.3. Testing the Integration
You can test the integration by sending a POST request to the /analyze-symptoms
endpoint with the patient's symptoms as the request body. The API will generate a medical advice response based on the symptoms provided, and you can evaluate the accuracy and relevance of the advice.
If the message of the patient would be: "I have a headache and a sore throat.", the response from the API could be:
{
"introduction": "Thank you for sharing your symptoms. Based on the information you provided, here are some possible conditions, treatments, and recommendations to consider.",
"possible_conditions": [
{
"condition": "Common Cold",
"confidence": 0.75,
"description": "A common viral infection of your nose and throat. Symptoms typically include a sore throat and a mild headache."
},
{
"condition": "Flu",
"confidence": 0.25,
"description": "An infection caused by the influenza virus that can affect your nose, throat, and sometimes your lungs. It often presents with a sore throat, severe headache, and other systemic symptoms."
}
],
"recommended_treatments": [
{
"treatment": "Over-the-counter Pain Relief",
"medications": [
{
"name": "Ibuprofen",
"dosage": "200 mg every 4 to 6 hours as needed",
"precautions": "Do not exceed 1200 mg in 24 hours unless directed by a healthcare provider."
},
{
"name": "Acetaminophen",
"dosage": "500 mg every 4 to 6 hours as needed",
"precautions": "Avoid if you have liver disease or consume three or more alcoholic drinks per day."
}
],
"instructions": "Take these medications with food to reduce stomach upset."
},
{
"treatment": "Home Care",
"medications": [],
"instructions": "Stay hydrated, rest, and use a humidifier to ease a sore throat and sinus congestion."
}
],
"advice": "Monitor your symptoms closely. If they worsen or if you experience high fever, difficulty breathing, or inability to eat or drink, seek medical attention immediately.",
"follow_up": {
"questions": [
"Do you have any allergies to medications?",
"Have you experienced similar symptoms in the past?",
"Are there any other symptoms, like fever or cough?"
],
"instructions": "Please answer these follow-up questions to help us better understand your condition and refine your treatment recommendations."
},
"additional_resources": [
{
"condition": "Common Cold",
"url": "https://www.mayoclinic.org/diseases-conditions/common-cold/symptoms-causes/syc-20351605"
},
{
"condition": "Flu",
"url": "https://www.cdc.gov/flu/symptoms/symptoms.htm"
}
],
"closing": "We hope this information helps you manage your symptoms effectively. Please keep us updated on your condition, and do not hesitate to contact a healthcare provider if you have any concerns."
}
This response provides a detailed medical advice based on the patient's symptoms, including possible conditions, recommended treatments, advice, follow-up instructions, and additional resources for further information.
The doctor would review the response, confirm the diagnosis or suggest further tests, and provide personalized care based on the patient's condition.
If the openAI diagnosis was correct, we would save a lot of time for the doctor and the patient would get the response immediately.
Conclusion
OpenAI's API offers a transformative potential across industries, capable of powering applications that significantly enhance user experiences and operational efficiencies. By understanding its capabilities and strategically integrating AI, businesses can unlock new avenues for innovation and growth.
Whether it's creating more engaging learning environments, providing faster medical assessments, or enhancing customer interaction, the possibilities with OpenAI's API are only limited by the imagination. As AI continues to evolve, its integration into applications will become more prevalent, reshaping how businesses interact with their customers and manage their operations.