Revolutionize Your E-Commerce using Google Apps Script & OpenAI
by Andrej Avbelj, Software Engineer
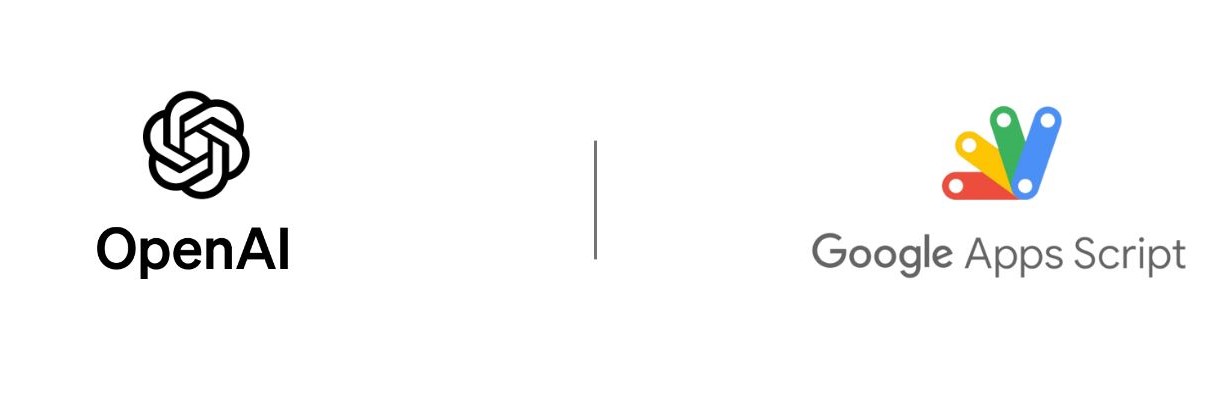
1. Introduction
Google Apps Script is a robust cloud-based scripting language based on JavaScript that allows you to automate tasks across Google products. In this blog post, we'll explore an advanced use case: generating product descriptions using OpenAI's API, then translating those descriptions into Spanish, German, and French using Google Apps Script's built-in LanguageApp service.
This example is particularly useful for e-commerce platforms or online stores looking to automate and internationalize their product listings. By the end of this guide, you will be able to fetch product metadata, generate descriptions in English, and then translate those descriptions into multiple languages, all within a Google Sheet environment.
2. Setting Up Your Google Sheet
Begin by preparing a Google Sheet with your product information. At a minimum, you should have columns for Product ID
, Product Name
, and Metadata URL
. Optionally, you can include a column for Product Image URL
if you plan to extend this functionality to include image processing.
From your Google Sheet, navigate to Extensions > Apps Script
to open the script editor. This is where you'll write the automation script.
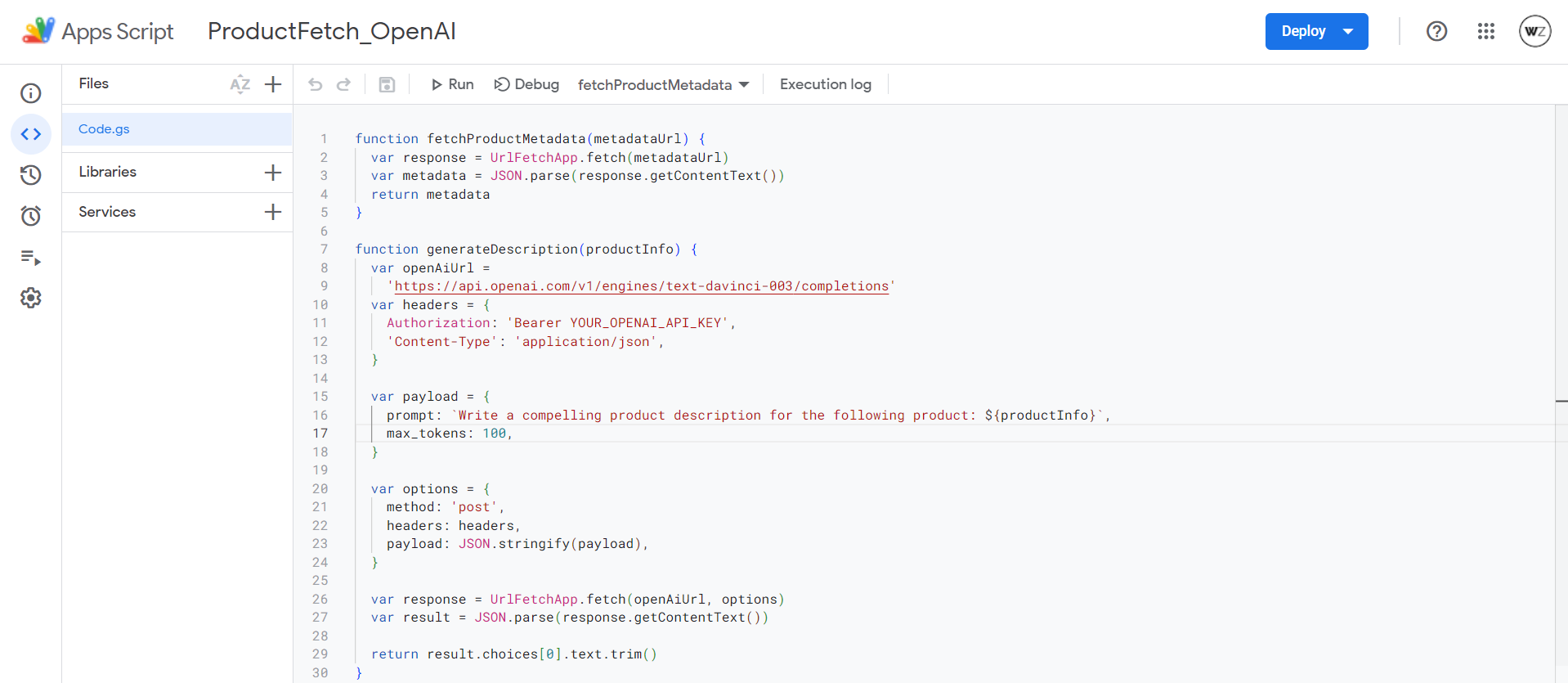
3. Fetching Product Metadata
Use Google Apps Script's UrlFetchApp
service to retrieve product metadata from an external API. The metadata might include details like price, dimensions, materials, etc., which can enrich the product descriptions.
function fetchProductMetadata(metadataUrl) {
var response = UrlFetchApp.fetch(metadataUrl)
var metadata = JSON.parse(response.getContentText())
return metadata
}
4. Generating Product Descriptions with OpenAI
To utilize OpenAI's API, ensure you have an API key from OpenAI. The following function takes product information, calls OpenAI's API, and generates an English description.
function generateDescription(productInfo) {
var openAiUrl =
'https://api.openai.com/v1/engines/text-davinci-003/completions'
var headers = {
Authorization: 'Bearer YOUR_OPENAI_API_KEY',
'Content-Type': 'application/json',
}
var payload = {
prompt: `Write a compelling product description for the following product: ${productInfo}`,
max_tokens: 100,
}
var options = {
method: 'post',
headers: headers,
payload: JSON.stringify(payload),
}
var response = UrlFetchApp.fetch(openAiUrl, options)
var result = JSON.parse(response.getContentText())
return result.choices[0].text.trim()
}
5. Translating Descriptions
After generating the English descriptions, use Google Apps Script's LanguageApp to translate them into Spanish, German, and French.
function translateDescription(description, targetLanguage) {
return LanguageApp.translate(description, 'en', targetLanguage)
}
6. Integrating Everything
Combine all steps in a main function that iterates over each product in your sheet, fetches metadata, generates an English description, and translates it.
function updateProductDescriptionsWithTranslations() {
var sheet = SpreadsheetApp.getActiveSpreadsheet().getActiveSheet()
var range = sheet.getDataRange()
var values = range.getValues()
for (var i = 1; i < values.length; i++) {
var row = values[i]
var metadata = fetchProductMetadata(row[2]) // Assuming URL in third column
var productInfo = `${row[1]} - ${JSON.stringify(metadata)}` // Product Name and Metadata
var description = generateDescription(productInfo)
// Translate the description
var descriptionSpanish = translateDescription(description, 'es')
var descriptionGerman = translateDescription(description, 'de')
var descriptionFrench = translateDescription(description, 'fr')
// Write descriptions back to the sheet
sheet.getRange(i + 1, 4).setValue(description) // Original in fourth column
sheet.getRange(i + 1, 5).setValue(descriptionSpanish) // Spanish in fifth
sheet.getRange(i + 1, 6).setValue(descriptionGerman) // German in sixth
sheet.getRange(i + 1, 7).setValue(descriptionFrench) // French in seventh
}
}
Conclusion
This solution showcases the power of combining Google Apps Script with external APIs like OpenAI to automate and internationalize e-commerce content. While the translation provided by Google's LanguageApp is a powerful tool, it's always a good idea to review translations for accuracy. Additionally, remember to monitor API usage to avoid exceeding rate limits.
By implementing this script, you can significantly streamline your product description workflow, making your products more accessible to a global audience. Happy scripting!